Learn Golang – 8 Ways To Master The Go Programming Language
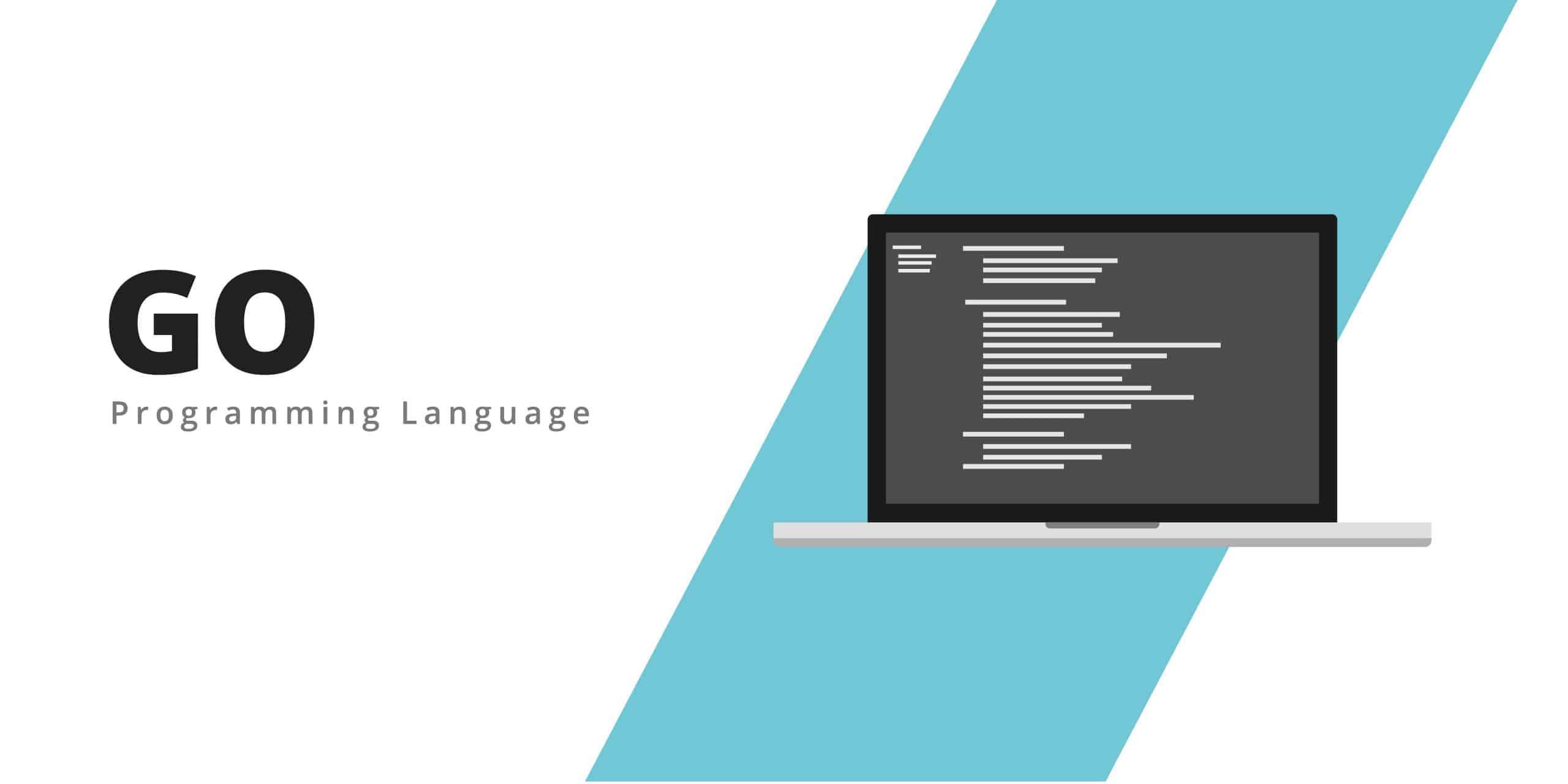
If you’ve read some of our previous blogs, you won’t be surprised when we say Golang is the best programming language to learn for new and experienced coders alike.
Golang offers the ease of use, clarity, and practicality that many other languages lack, and it’s relatively simple to pick up.
All that’s left now is to master it, and today’s your lucky day – cos we’ve got the cheat sheet!
Whether you’re a seasoned pro with multiple programming languages under your belt, or a relative novice just starting out, there really is no reason why you shouldn’t be learning it.
Go stands a little apart from other languages in that it’s idiomatic. It’s not just about compiling the code, it’s about writing it with the Golang mindset, in the Golang way.
Think of it a little like learning chess. If you’ve never played the game before, you have to get a handle on the rules and tactics before you’re able to jump in and participate in a match. Just because you know how to play backgammon and checkers, doesn’t mean you have some natural talent or innate ability when it comes to chess.
There are a bunch of online resources at your fingertips, ranging all the way from professionally produced, to reddit questions and answers – with a whole kaleidoscope of how-to’s, cheat sheets, and templates in between. It can be quite daunting, and a case of “where the hell do I start?”
Thankfully, we’ve put together 8 ways to master the Go programming language that are helpful for everyone from novices to senior programmers, and although these methods may be obvious to some people – they may not be as obvious to others.
1 – GOING STRAIGHT TO THE SOURCE:
The first point of call must be to search out and complete Google’s own learn Golang course “A Tour of Go”. Both beginner and experienced programmers will benefit a lot from starting from scratch and getting across the basics.
The course is run by Todd McLeod, arguably one of the most passionate and knowledgeable teachers of Golang out there. He’s a professor of computer science at ‘California State University Fresno’ and has also tenured at ‘Fresno City College’ – after more than 2 decades of teaching – the man knows his stuff.
The most important decision to make is to learn from the best and decide on a teacher that suits you.
2 – TRUST THE SYSTEM:
Let’s take a step back for a minute and take a look at the why and the how. In 2006 Google, hands down an industry leader in software engineering, decided that none of the programming languages were meeting their needs and doing exactly what they wanted them to do.
The solution? To make their own. That’s huge! Take a look at the credentials of Golang for a minute – its open source, and it was created by the dream team. We’re talking the absolute superstars of the computer science world. The ‘Kobe Bryants’ and the ‘Tom Bradys’ – the MVPs of programming.
Google hired ‘Robert Griesemer’, ‘Rob Pike’, and ‘Ken Thompson’. These were the guys who helped created the C programming language, the UNIX operating system, and the UTF-8 encoding scheme which is the most popular coding scheme in the world today.
Google had 3 main goals with Golang – efficient compilation, efficient execution, and ease of programming. When the open source went live in 2012, no programming language around at that time could nail more than 2 out of these 3 desirable traits.
The key point here is ease of programming – to learn Golang, it’s designed to be easy as simplicity is a feature. A seamless garbage collector, built in server packages, incorporated concurrency management and circular dependency detection are all features designed to make sure you have a good time learning Golang, and not a bad time.
3 – STEP IT UP A NOTCH:
After taking “A Tour of Go”, the next step would be to go to golang.org and soak up all the wisdom contained in “Effective Go”. Think of it as the intermediate program that beginners graduate to after they’re learnt about the fundamentals.
There are handy examples of how each function is used, how to write it in code, and why certain syntax is used. C and C++ programmers may have a distinct advantage here and at least some of the Golang content would look very familiar.
4 – USE THE RIGHT TOOLS:
Just know that ‘Gofmt’ is your friend. It’s a tool that automatically formats Golang source code so it’s even easier to write, as minor formatting errors won’t create as many bugs. 70% of Golang uses ‘Gofmt’, so the need to mentally convert the formatting style of someone else to your own format style is a thing of the past. Maintenance is a breeze as mechanical changes to the source code don’t change the formatting of the files.
You’ll never have to debate about spacing or brace position – ever. Use ‘Gofmt’ from the very start and reap the benefits right off the bat.
5 – INTERFACES FOR THE WIN:
If one thing sticks in your mind, it should be this – define interfaces for your types whenever you can.
Interfaces in Golang are kind of like definitions. They describe and define the exact methods that some other type must have. Whenever you see a declaration in Golang, such as a variable, function parameter, or structure field, so long as it has an interface type – the interface can be satisfied by another object of any type.
This helps reduce duplication or boilerplate style coding where terms are repeated again and again. The added benefit is that mock-ups can be used in lieu of real objects in unit tests. Also, using this architecture enforces decoupling between the various parts of your codebase.
6 – THERE’S NO MAJORITY RULE:
Channels are great as they provide built-in thread safety and encourage single threaded access to shared critical resources in a system, however they come with a performance penalty. Think of them as the pipes that connect concurrently running ‘GoRoutines’.
You can send values into channels from one ‘GoRoutine’ and input them into another. This is best used when passing ownership of data, distributing units of work, or communicating async results.
However, channels aren’t gospel. Sometimes using good old Mutex is the way to go. It’s particularly helpful when you just need locks over a few shared resources, to guard the internal state of a structure, or if performance is a critical aspect of your algorithm.
7 – ALWAYS CHECK:
Before you start hammering away at a Golang package for anything, make sure you check and understand whether that package supports concurrency or not before you start firing away ‘GoRoutines’.
It’s important that the functions can run independent of each other. The Golang runtime scheduler actually has a feature that manages all the ‘GoRoutines’ that are created and require the use of a processor. This scheduler then binds the operating system’s threads to logical processors so they can execute.
Also, don’t ever use the unsafe package unless you absolutely understand what you’re getting yourself into and are confident working within its confines. There are operations here that sidestep the type safety of Go programming, and are not protected by the compatibility guidelines. It’s all there in the name – unsafe.
8 – TURN THE TABLES:
Consider using table driven tests in Golang. Testing is something that beginner level programmers seem to neglect – it’s a weird phenomenon. You write the code but avoid testing it at all costs – despite the fact that it may contain duplicate code and may be missing edge cases.
Embrace the D.R.Y or Don’t Repeat Yourself principle. However, testing can be tedious, so there are some clever tricks to make testing more fun – not to mention easier.
Table driven tests cover much more ground, as each table entry is a complete test case with inputs and expected results. The test itself just iterates through all the table entries and performs the necessary tests for each. The table itself provides the inputs and expected results of the function that is being tested.
The Best Thing You Can Do
The only way to really get better at learning ‘Golang’ is to jump in and start writing. Try finding a project that has some “help needed” issues flagged and open collaboration, then get in there and make a contribution.
Find out if some of the bugs require beginner level solutions and make your first pull request – after all, the only way to improve is through experience and by practicing.
Learn by building, rather than learning all the rules out of a book.
How To Learn and Master Golang
Where are the best places to begin learning Golang? We’ve carefully sifted through the plethora of online courses and tutorials and reduced it down to a mere handful. In our opinion, these are the 5 best online resources to learn Golang – a must read for beginners and advanced programmers alike.
1 – Learn How to Code: Google’s Go (Golang) Programming Language:
Udemy offers the ultimate comprehensive course that covers Go from head to toe. So far, over 2.65 million students have completed the course, which contains expert tutelage from university professor and Golang genius Todd McLeod, as well as hands-on exercises with solutions.
Definitely worth a look, and learn at your own pace – as fast or as slow as you need to.
2 – Go: The Complete Developer’s Guide (Golang):
This is another Udemy based course where you can learn both the fundamentals and the more advanced features of Golang. This one is presented by Stephen Grider, who is well accomplished in the industry and focuses more on concurrency and using interface type programming systems.
Well worth the investment.
3 – Programming with Google Go Specialization:
This Coursera offering covers the basics and concurrency, but really places a big focus and emphasis on the functions, methods, and interface used in Go. The added benefit is you get a nifty certificate of completion which looks great included in a resume.
This is a go-to source if you want to create clean, efficient applications.
4 – Go Fundamentals by Nigel Poulton:
Pluralsight has a course that is kinda like “Go For Dummies”. It assumes you have zero prior knowledge, and starts from the very, very basics – and builds your knowledge from the ground up.
This is probably one of the best resources for absolute newbies and novices.
5 – Learn Go:
Codecademy is possibly one of the best hubs for interactive online learning. It has a whole heap of courses to cover other programming languages like Python, Bash and JavaScript but also has a handy Go course as well. They’re partnered with Google – the creators of Golang.
This is a great resource for people who learn better from doing, instead of just reading.
Put Your Skills Into Action
Any of these 5 online courses can get you what you need to get started mastering Golang. If you’re looking to make a career move and become a Golang developer contact Kofi Group to learn more about the demand in this field and how to make that transition.
For more on Golang check out our previous blog post “5 Reasons Why Go Is the Best Programming Language to Learn in 2021” and check out where it stacks up compared to the reigning top languages in “The Top 10 Programming Languages of All Time”.
Kofi Group is proud to be a source of knowledge and insight into the startup software engineering world and offers a multitude of resources to help you learn more, improve your career, and help startups hire the best talent. If you are interested in learning more about what we do and how we can help you then get in touch or watch our Youtube videos for additional information.
Share This Blog
Kofi Group has helped 100+ startups hire software and machine learning engineers. Will fill most of the roles we recruit on with 5 or less candidates presented.
Contact us today to start building your dream team!