Node.JS and 5 Things You Need to Know About it in 2021
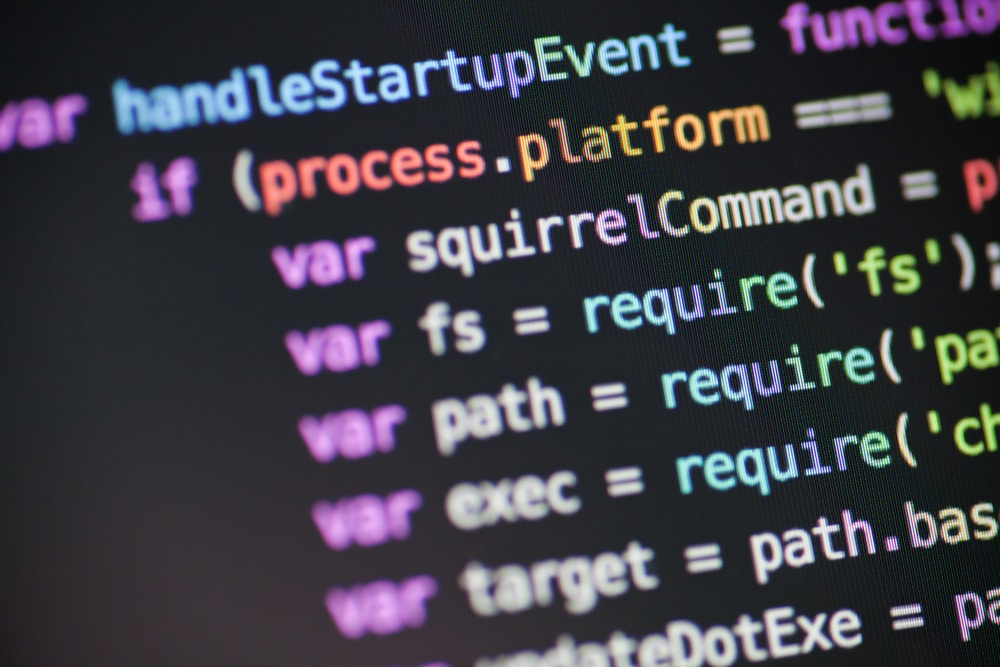
Who likes to go out to a restaurant to eat every once and a while?
It’s nice to treat your significant other or buddies to a restaurant meal every now and then, but the idea soon becomes a bad one if the service is slow or lagging – you end up with a table full of grumpy, empty-bellied guests who eventually threaten to leave.
Just like hungry guests who have waited too long can leave the restaurant, requests can “time out” if the server takes too long to process them.
So how can we make sure queries don’t get hangry waiting for their JavaScript?
Enter Node.JS and the 5 things you need to know about it in 2021!
NODE.JS 101
We always try and use an analogy for the Layperson, but this week’s one is a doozy – so pay attention. The restaurant metaphor is actually a pretty good way to explain the relationship between the server and JavaScript requests, but also goes further to explain how and why Node.js performs so much better than regular JavaScript on the server-side.
Everyone’s actually eaten at a restaurant, right? A customer walks in, and they’re seated by a waiter who takes their order and notifies the kitchen to prepare the meal – before bringing it out to them when it’s ready.
Once the order (request) is taken from the customer (client) by the waiter (event loop), he takes the order to the kitchen pass (event queue) where it’s assigned to a chef (callback). Think of the chef as the access to the files on the server, who controls the food storeroom (input), as well as which ingredients and portion sizes make up the dish (output).
However, ordering food supplies is ultimately the responsibility of the restaurant owner (the operating system). The waiter (event loop) constantly checks the kitchen pass (event queue) to see if the order (request) is ready, so they can then take it to the customer’s (client’s) table.
Now, this is exactly how JavaScript requests were processed, but crazily, there was one waiter assigned to each customer that could do nothing except wait for the meal to be ready. Node.js changes this up by allowing the waiter to take more orders from other guests while they wait for a call from the kitchen for table service. By having the event loop able to handle multiple threads at once, all of a sudden, a server lacking in system resources is still able to handle a pretty large number of requests in a timely manner.
If the customer orders right away, they take up hardly any of the waiter’s time so they can get right onto serving the next customer – just like non-blocking code that doesn’t increase the processing interval. But say that customer wants to ask the waiter about a particular dish, or to add something to an item on the menu, then this would require the waiter’s attention for a few more minutes – this is blocking code, a request that can only be processed once all the variables related to the request have been confirmed with the client. Think of it like an especially large file that needs to be read, with other tasks to be executed afterwards.
Likewise, packets of cutlery and pre-folded napkins drastically reduce the time the waiter needs to prepare the table and give the customer everything they need to eat their meal. In Node.js these are Node Packaging Managers (NPMs), a library of pre-written bits of code that can be installed to perform menial tasks without the need to write them from scratch.
But what about if you hire a general manager to oversee the day to day running of the restaurant, and makes sure that things run smoothly and all customers are served in both a timely fashion, and are happy with their meals? You’d be looking at Express.js.
Sure, the waiter takes care of the order, service, and paying of the cheque, but it’s the general manager who’s making other decisions like if the customer is adequately dressed to dine at the restaurant, or if customers are over 21 and can be served alcohol – just the same as Express.js handles things like login credentials and whether the request comes from an actual account with the correct password.
The customer prefers to order appetizers, main course, desert, and coffee all at the same time, so both the waiter and the chef need some guidance from the general manager of the restaurant to help with the timing of the courses. This is exactly how Express.js functions as a layer of middleware within Node.js that creates that little bit of abstraction for the client user to issue customized requests to the server.
The general manager would also be able to make judgement calls on whether to seat people at the bar, inside at a table, or on the outdoor terrace based on how many people are in the party, whether they’re ordering main courses or light appetizers while having a glass of wine.
Node.js which would just have a single waiter serving all customers, scaling poorly as demand rises. Even so, that waiter is the best in the world, and easily outworks 5 regular waiters – just how Node.js is often more efficient than other multi-threaded systems with just a single thread.
The Express.js layer can hire more wait staff and more chefs in the same way that it allows Node.js to become multi-threaded, even if it is somewhat restricted with numbers.
1- What Is Node.js:
Node.js is a JavaScript runtime environment that performs everything needed to execute a program written in JavaScript. It came into being when JavaScript evolved from something purely browser based to something that could be run on a system as a standalone application tasked with back-end server request management.
Basically, Express.js adds a set of rules that need to be applied and satisfied before any requests can be made to the server.
2-What Node.js is Used For:
Before Node.js, web-based apps incorporated a client/server model – simple enough, the client would request resources from the server, and the server would respond by providing the client with those resources. Each request was allocated processing and memory, and once completed, the connection was closed to free up those system resources to complete a different request etc.
Node.js adds utility by allowing the event loops to handle multiple requests while they wait for the callback to dump the information in the event queue for delivery to the client. Node.js is built on Chrome’s VS JavaScript engine, and uses a non-blocking, event driven input and output model so that it doesn’t get bogged down in the detail – making it extremely lightweight and efficient. It’s also backed by one of the largest collections of open-source libraries in the world, covering a very wide range of uses.
3- Pros:
Non-Blocking Code:
Being completely event driven and running code based on callbacks from the event loop system, Node.js is available to perform other requests while waiting for previous ones to be completed without causing much of an increase to the processing delay of the event queue.
It’s Fast:
Node.js is based off the Chrome V8 Runtime engine, which is the same one the Chrome browser uses. Both Node.js and V8 are written in C programming language, which makes it super quick – much faster than others like Ruby or Python.
True Concurrency:
Node.js can cope with thousands of concurrent connections and requests as a single process thread, which isn’t very resource hungry and needs very minimal overheads from what may be a resource poor system with aging server hardware.
One Environment:
Using JavaScript on both the front and back end of an application has some pretty distinct advantages, the main one being the elimination of miscommunication between two different programming environments. Node.js communicates with client-side JavaScript without needing JavaScript Object Notation (JSON) as they have a common syntax.
Easy To Learn:
Being JavaScript based, Node.js has little to no learning curve for those programmers already proficient, but also, Node.js is super easy for relative coding novices to pick up and is one of the more forgiving programming languages out there. Besides, JavaScript is a popular language with huge community support – so there’s an abundance of knowledgeable people to ask for solutions to any issues you encounter.
4- Cons:
Limited Scalability:
Remember how Node.js just has the single, awesome waiter? Well, a single CPU core has a limit to how many requests it can handle at a time, so unless you have an Express.js layer, Node.js won’t make full use of system resources like a multi-core CPU – which is the norm in even the most basic home computers, let alone big server racks. Then again that single thread does out-perform a lot of multi-threaded back ends.
Relational Databases:
Node.js hates relational databases, we thoroughly recommend you never mix the two as they go together just about as well as oil and water.
However, we will add that there is an argument that you can use relational databases with node.js but the main issue is that it isn’t popular within the community so you would lack support in your endeavors. For the brave soul feel free to venture out and try it, but we warn you it may be a laborious task.
Nested Callbacks:
It turns out that JavaScript can do some weird stuff, and you can get nested callbacks – which are callbacks, stuck in a callback, that is stuck in another callback. This can quickly create an exponential backlog in the event queue and suck up some serious computational power.
Stick To the Server:
Node.js is best suited for something like a web server, where it has to manage the input and output of stored data. Node.js isn’t great at CPU-intensive tasks because it itself is CPU-intensive by its very nature.
5- Which Companies Are Using Node.js:
It’s not surprising to see a whole bunch of highly successful and instantly recognizable companies using Node.js, with a real list of who’s who across multiple industries that includes LinkedIn, Netflix, Uber, Trello, PayPal, NASA, eBay, Medium, Groupon, Walmart… the list is as long as your leg really.
These companies run Node.js as the preferred runtime environment for servers associated with JavaScript application development.
Node.js is something that really broke the mold, a reaction to the snail’s pace that classic JavaScript operated at when resolving client requests for information stored on the server. The callback concept and the ability for the event loop to line up multiple requests in a queue meant that a single thread could essentially take on a whole ton of work with minimal delays, compared to a multi-threaded system where a thread’s query had to be resolved before another could be taken.
JavaScript’s popularity surpassed the browser, and infiltrated the unseen art of backend programming and management in the form of Node.js, and with Express.js adding even more utility to the runtime environment, that popularity is growing by the day – culminating in Node.js being adopted by some seriously successful companies.
HOW TO GET STARTED?
Node.js is a solid pick for those with web-based application background in JavaScript looking to make the shift to back-end programming, and also for aspiring programmers wanting some decent tools at their disposal.
1 – The Complete Node.js Developer Course:
Arguably the most all-encompassing Node.js resource on the web, and the go to for all novices.
2 – Node.js – The Complete Guide:
Another beginner friendly course that also covers more advanced concepts in more detail making it great for intermediate and expert level JavaScript coders.
3 – Node.js Advanced Concepts:
Now we’re getting somewhere. If you’re a wizard at JavaScript, then this course is for you as it focuses on the real nitty gritty of Node.js.
4 – Node.js, Express, MongoDB & More: The Complete Bootcamp 2021:
This is the course to complete if you want to know more about how Express.js layers across Node.js, and how they function with other database systems.
5 – Introduction to Node.js:
This course is a little different in that it focuses on the strategy behind using Node.js to modularize your applications.
Next Steps
If you enjoyed this blog and are interested in all things JavaScript related then check out our blogs on Next JS and 7 Things You Need to Know About It and P5JS and 5 Things You Need to Know About It.
Kofi Group is proud to be a source of knowledge and insight into the startup software engineering world and offers a multitude of resources to help you learn more, improve your career, and help startups hire the best talent. If you are interested in learning more about what we do and how we can help you then get in touch or watch our Youtube videos for additional information.
Share This Blog
Kofi Group has helped 100+ startups hire software and machine learning engineers. Will fill most of the roles we recruit on with 5 or less candidates presented.
Contact us today to start building your dream team!